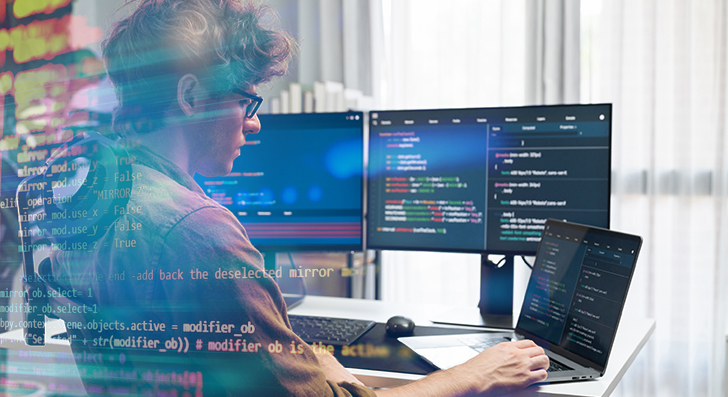
Scalability implies your software can take care of progress—much more buyers, additional knowledge, and even more visitors—without breaking. To be a developer, constructing with scalability in mind saves time and strain later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't one thing you bolt on afterwards—it should be part of your respective strategy from the start. Numerous purposes fail every time they expand speedy since the first design and style can’t cope with the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Instead, use modular design and style or microservices. These styles crack your app into smaller sized, impartial pieces. Every module or provider can scale By itself with out impacting The full procedure.
Also, consider your database from day one particular. Will it have to have to handle a million consumers or perhaps 100? Select the ideal type—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them nevertheless.
A different important point is to prevent hardcoding assumptions. Don’t compose code that only operates beneath recent ailments. Contemplate what would take place When your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use structure styles that aid scaling, like information queues or occasion-driven systems. These help your application tackle additional requests devoid of finding overloaded.
Any time you Create with scalability in your mind, you are not just getting ready for success—you're lessening long run complications. A effectively-planned system is less complicated to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the right databases can be a essential Portion of developing scalable purposes. Not all databases are created the identical, and using the Erroneous one can gradual you down or perhaps induce failures as your app grows.
Start out by knowing your data. Can it be very structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is a good healthy. These are sturdy with relationships, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to deal with additional site visitors and details.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with huge volumes of unstructured or semi-structured facts and can scale horizontally far more easily.
Also, contemplate your browse and create designs. Are you carrying out numerous reads with less writes? Use caching and skim replicas. Are you handling a weighty generate load? Consider databases that could cope with high create throughput, as well as celebration-based mostly facts storage units like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not have to have Sophisticated scaling functions now, but deciding on a databases that supports them means you won’t need to switch later.
Use indexing to speed up queries. Stay away from unwanted joins. Normalize or denormalize your details based on your accessibility styles. And generally monitor databases performance as you develop.
In brief, the correct databases is dependent upon your application’s framework, velocity desires, And exactly how you be expecting it to improve. Acquire time to choose properly—it’ll conserve plenty of problems later.
Improve Code and Queries
Quick code is key to scalability. As your application grows, each individual smaller hold off adds up. Poorly created code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct successful logic from the start.
Begin by crafting cleanse, uncomplicated code. Stay away from repeating logic and remove anything avoidable. Don’t select the most complicated Alternative if a straightforward just one operates. Keep your features brief, concentrated, and simple to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes way too lengthy to operate or makes use of too much memory.
Following, take a look at your databases queries. These frequently sluggish issues down in excess of the code itself. Ensure that Just about every query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Specially throughout big tables.
When you notice the identical details becoming requested time and again, use caching. Store the outcome briefly applying equipment like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your databases functions whenever you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more productive.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code limited, your queries lean, and use caching when needed. These actions assist your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and much more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted visitors throughout many servers. As an alternative to one particular server carrying out all of the function, the load balancer routes users to distinctive servers based on availability. Gustavo Woltmann blog This suggests no solitary server gets overloaded. If one server goes down, the load balancer can send out traffic to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing data quickly so it may be reused quickly. When buyers request the same information and facts once again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve generally. And always be sure your cache is updated when info does improve.
In brief, load balancing and caching are uncomplicated but powerful equipment. Together, they help your application handle a lot more people, stay quickly, and recover from difficulties. If you intend to mature, you will need equally.
Use Cloud and Container Applications
To construct scalable programs, you require applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you overall flexibility, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess potential capability. When targeted traffic boosts, you may add much more sources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and safety tools. You can focus on building your application in place of taking care of infrastructure.
Containers are A further critical Resource. A container deals your app and all the things it really should operate—code, libraries, settings—into one device. This can make it effortless to move your application involving environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked Device for this.
When your application employs numerous containers, applications like Kubernetes make it easier to control them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature without having restrictions, begin employing these resources early. They help you save time, decrease chance, and help you remain centered on building, not repairing.
Watch Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your application is accomplishing, spot troubles early, and make improved decisions as your app grows. It’s a essential Component of building scalable methods.
Start off by monitoring primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Keep an eye on how long it takes for customers to load webpages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or maybe a assistance goes down, it is best to get notified quickly. This will help you take care of difficulties rapid, typically just before consumers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new attribute and see a spike in errors or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, website traffic and info improve. Without the need of checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking aids you keep your app reliable and scalable. It’s not almost recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for major organizations. Even smaller apps need a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you may Develop apps that mature easily with no breaking under pressure. Commence smaller, think massive, and Establish intelligent.